Automate your notification system with Python
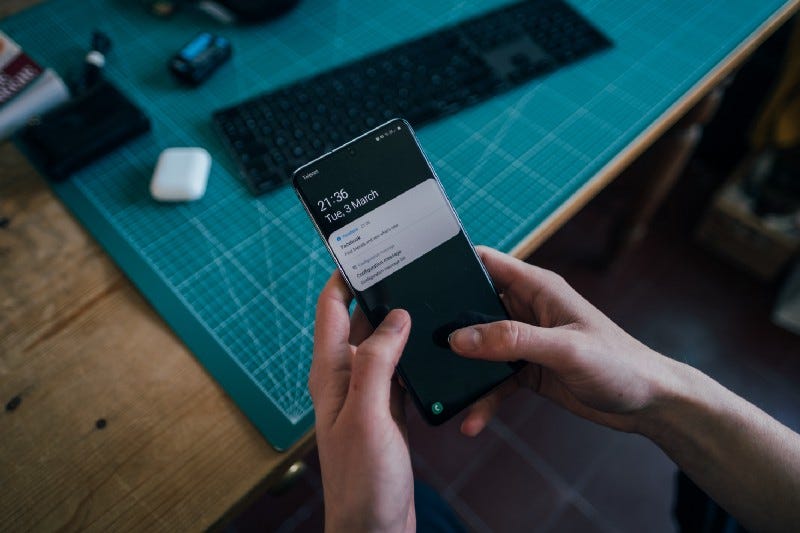
Have you been in a position where you are waiting for your machine learning model and need to go somewhere? Or you in the middle of another task and continuously thinking if your script has successfully run or not? I have been in that position before, and that is why I am creating an automatic notification system to my email using Python.
In this article, I would explain how to create the automation system with Python in a few simple steps. Let’s just get into it.
Email Preparation
For simplicity reason, I would use an email system from Gmail. What we need to do first is setting up the Gmail API from the Google API Console. In the developer dashboard, select the ENABLE APIS AND SERVICES.
From there, try type and search the Gmail API.
In the Gmail API selection, click ENABLE to activate our Gmail API.
When it’s done, we need to create credentials in to access the email and send the notification using Gmail.
Select the OAuth client ID, and when asked for the Application Type, as I am using Jupyter Notebook to run my script, I would choose the Desktop App.
Name it to whatever you like, here I just called it gmail_python. When you finish creating the credentials, you need to download it in the JSON form. For my recommendation, it is better to rename it as ‘credentials.json’.
Place the file in the same folder with your Jupyter Notebook (or script).
Creating the Notification System
The email system would be based on the recommendation given by the Google Gmail API to send an email. I would also use the email module to help me build the system for sending an email.
First, let’s create our function to generate the message.
from email import encodersfrom email.mime.base import MIMEBasefrom email.mime.multipart import MIMEMultipartfrom email.mime.text import MIMETextimport base64import os
def create_message(sender, to, subject, message_text): """Create a message for an email.
Args: sender: Email address of the sender. to: Email address of the receiver. subject: The subject of the email message. message_text: The text of the email message.
Returns: An object containing a base64url encoded email object. """ message = MIMEText(message_text) message['to'] = to message['from'] = sender message['subject'] = subject return {'raw': base64.urlsafe_b64encode(message.as_string().encode()).decode()}
Then we create the function to send the message.
def send_message(service, user_id, message): """Send an email message.
Args: service: Authorized Gmail API service instance. user_id: User's email address. The special value "me" can be used to indicate the authenticated user. message: Message to be sent.
Returns: Sent Message. """ try: message = (service.users().messages().send(userId=user_id, body=message) .execute()) print('Message Id: {}'.format(message['id'])) return message except: print ('An error occurred')
Now we have two functions to create the message and sending the email. For the next step, let’s create a function to automate the notification system. Here we need the previously created credentials from the google Gmail API. In here, I set up my system to use the credential JSON file as ‘credentials.json’.
def notification(sender, to, subject, notification):
#Sender is the sender email, to is the receiver email, subject is the email subject, and notification is the email body message. All the text is str object.
SCOPES = 'https://mail.google.com/' message = create_message(sender, to, subject, notification) creds = None
if os.path.exists('token.pickle'): with open('token.pickle', 'rb') as token: creds = pickle.load(token) #We use login if no valid credentials if not creds or not creds.valid: if creds and creds.expired and creds.refresh_token: creds.refresh(Request()) else: flow = InstalledAppFlow.from_client_secrets_file('credentials.json', SCOPES) creds = flow.run_local_server(port=0) # Save the credentials for the next run with open('token.pickle', 'wb') as token: pickle.dump(creds, token)
service = build('gmail', 'v1', credentials=creds) send_message(service, sender, message)
With our notification function is ready, we can try to send the notification. For example, I would try to learn a machine learning model below.
import seaborn as snsimport pandas as pdfrom sklearn.linear_model import LinearRegression
#Loading the dataset and only using the numerical variablesmpg = sns.load_dataset('mpg').drop(['origin', 'name'], axis = 1)linear_model = LinearRegression()
try: #Training the model linear_model.fit(mpg.drop('mpg', axis =1), mpg['mpg'])
notification('test1@gmail.com', 'test2@gmail.com', 'Notification - Success Training', 'The model has finish')except: notification('test1@gmail.com', 'test2@gmail.com', 'Notification - Failed Training', 'The model encountered error')
Now I get my notification when the training is done or if they encountered an error. The above data we use for training containing NaN value, so it is obviously would lead to error. Although, my point is that we capable of creating the notification system to our email now.
Conclusion
In this article, I have shown you how to create a simple notification system to your email using python. You can modify the system as you like.